Learn how to evaluate and integrate the VNC SDK
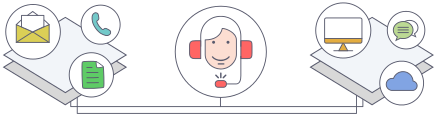
Server¶
-
class Server
¶ A VNC-compatible Server enabling a computer to be remotely controlled.
For more information, see
vnc_Server
in the C API documentation.
Implements¶
Nested classes¶
- Server.SecurityCallback
- Server.InputEventsCallback
- Server.ConnectionCallback
- Server.AgentCallback
- Server.DisconnectFlags
- Server.CaptureMethod
- Server.Permissions
- Server.EncryptionLevel
Constructor summary
Modifier and Type | Method and Description |
---|---|
Creates a Server. |
Method summary
Modifier and Type | Method and Description |
---|---|
static Server |
Creates a Server running as a service. |
void |
Destroys the Server. |
void |
Sets connection-related callbacks for the Server. |
Int32 |
Returns the total number of Viewers currently connected to the Server. |
void |
Sets security-related callbacks for the Server. |
void |
Changes permissions for a Viewer mid-session. |
Server.Permissions |
Gets the set of current permissions for a Viewer. |
void |
Sets agent-related callbacks for the Server. |
Boolean |
Determines if the |
void |
Specifies a friendly name for the Server, to send to connected Viewers. |
ConnectionHandler |
Obtains the Server’s |
String |
Returns the address of a particular connected Viewer. |
void |
Disconnects a particular Viewer, optionally specifying a message. |
void |
Disconnects all Viewers, optionally specifying a message. |
void |
Specifies a blacklist threshold and timeout for the Server. |
void |
Enables transmission of audio from the Server to the Viewer. |
AnnotationManager |
Obtains the Server’s |
MessagingManager |
Obtains the Server’s |
DisplayManager |
Obtains the Server’s |
void |
Sets the desired encryption level of the session from the range of options
enumerated by |
Server.EncryptionLevel |
Returns the encryption level being used with an incoming connection, or the Server’s current encryption level if the connection is null. |
void |
Set a password for use with third-party VNC Viewers, using VncAuth authentication. |
void |
Sets the number of seconds to wait before disconnecting idle Viewers. |
Int32 |
Gets the current number of seconds to wait before disconnecting idle Viewers. |
void |
Sets input control related callbacks for the Server. |
void |
Specifies the screen capture method used by the Server. |
Constructors¶
-
Server
(String agentPath)¶ Creates a Server.
For more information, see
vnc_Server_create()
in the C API documentation.
Methods¶
-
static Server
Server.CreateService
(String agentPath)¶ Creates a Server running as a service.
For more information, see
vnc_Server_createService()
in the C API documentation.
-
void
Server.Dispose
()¶ Destroys the Server.
For more information, see
vnc_Server_destroy()
in the C API documentation.
-
void
Server.SetConnectionCallback
(Server.ConnectionCallback callback)¶ Sets connection-related callbacks for the Server.
For more information, see
vnc_Server_setConnectionCallback()
in the C API documentation.
-
Int32
Server.GetConnectionCount
()¶ Returns the total number of Viewers currently connected to the Server.
For more information, see
vnc_Server_getConnectionCount()
in the C API documentation.
-
void
Server.SetSecurityCallback
(Server.SecurityCallback callback)¶ Sets security-related callbacks for the Server.
For more information, see
vnc_Server_setSecurityCallback()
in the C API documentation.
-
void
Server.SetPermissions
(Connection connection, Server.Permissions perms)¶ Changes permissions for a Viewer mid-session.
For more information, see
vnc_Server_setPermissions()
in the C API documentation.
-
Server.Permissions
Server.GetPermissions
(Connection connection)¶ Gets the set of current permissions for a Viewer.
For more information, see
vnc_Server_getPermissions()
in the C API documentation.
-
void
Server.SetAgentCallback
(Server.AgentCallback callback)¶ Sets agent-related callbacks for the Server.
For more information, see
vnc_Server_setAgentCallback()
in the C API documentation.
-
Boolean
Server.IsAgentReady
()¶ Determines if the
vncagent
process is ready and available to capture the display and inject input events.For more information, see
vnc_Server_isAgentReady()
in the C API documentation.
-
void
Server.SetFriendlyName
(String name)¶ Specifies a friendly name for the Server, to send to connected Viewers.
For more information, see
vnc_Server_setFriendlyName()
in the C API documentation.
-
ConnectionHandler
Server.GetConnectionHandler
()¶ Obtains the Server’s
ConnectionHandler
for performing connection operations.For more information, see
vnc_Server_getConnectionHandler()
in the C API documentation.
-
String
Server.GetPeerAddress
(Connection connection)¶ Returns the address of a particular connected Viewer.
For more information, see
vnc_Server_getPeerAddress()
in the C API documentation.
-
void
Server.Disconnect
(Connection connection, String message, Server.DisconnectFlags flags)¶ Disconnects a particular Viewer, optionally specifying a message.
For more information, see
vnc_Server_disconnect()
in the C API documentation.
-
void
Server.DisconnectAll
(String message, Server.DisconnectFlags flags)¶ Disconnects all Viewers, optionally specifying a message.
For more information, see
vnc_Server_disconnectAll()
in the C API documentation.
-
void
Server.SetBlacklist
(Int32 threshold, Int32 timeout)¶ Specifies a blacklist threshold and timeout for the Server.
For more information, see
vnc_Server_setBlacklist()
in the C API documentation.
-
void
Server.EnableAudio
(Boolean enable)¶ Enables transmission of audio from the Server to the Viewer.
For more information, see
vnc_Server_enableAudio()
in the C API documentation.
-
AnnotationManager
Server.GetAnnotationManager
()¶ Obtains the Server’s
AnnotationManager
for handling annotation operations.For more information, see
vnc_Server_getAnnotationManager()
in the C API documentation.
-
MessagingManager
Server.GetMessagingManager
()¶ Obtains the Server’s
MessagingManager
for handling messaging.For more information, see
vnc_Server_getMessagingManager()
in the C API documentation.
-
DisplayManager
Server.GetDisplayManager
()¶ Obtains the Server’s
DisplayManager
, for managing the list of displays made available by the Server and for setting the Server’s capture method.For more information, see
vnc_Server_getDisplayManager()
in the C API documentation.
-
void
Server.SetEncryptionLevel
(Server.EncryptionLevel level)¶ Sets the desired encryption level of the session from the range of options enumerated by
Server.EncryptionLevel
.For more information, see
vnc_Server_setEncryptionLevel()
in the C API documentation.
-
Server.EncryptionLevel
Server.GetEncryptionLevel
(Connection connection)¶ Returns the encryption level being used with an incoming connection, or the Server’s current encryption level if the connection is null.
For more information, see
vnc_Server_getEncryptionLevel()
in the C API documentation.
-
void
Server.SetVncAuthPassword
(String password)¶ Set a password for use with third-party VNC Viewers, using VncAuth authentication.
For more information, see
vnc_Server_setVncAuthPassword()
in the C API documentation.
-
void
Server.SetIdleTimeout
(Int32 idleTimeout)¶ Sets the number of seconds to wait before disconnecting idle Viewers.
For more information, see
vnc_Server_setIdleTimeout()
in the C API documentation.
-
Int32
Server.GetIdleTimeout
()¶ Gets the current number of seconds to wait before disconnecting idle Viewers.
For more information, see
vnc_Server_getIdleTimeout()
in the C API documentation.
-
void
Server.SetInputEventsCallback
(Server.InputEventsCallback callback)¶ Sets input control related callbacks for the Server.
For more information, see
vnc_Server_setInputEventsCallback()
in the C API documentation.
-
void
Server.SetCaptureMethod
(Server.CaptureMethod captureMethod)¶ Specifies the screen capture method used by the Server.
For more information, see
vnc_Server_setCaptureMethod()
in the C API documentation.